Translate
Tuesday 10 July 2018
Friday 15 September 2017
Thursday 14 September 2017
Core Java Tutorial Home

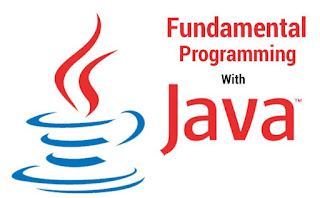
Hi guys hope you are doing great. If you really want to be master in core java concepts please follow this tutorial step by step. I promise if you will follow this on regular basis you will be very good in core java. After each tutorial I will provide you some practice questions. Please try to solve them as it will be the best way to remember those concepts.
So I will divide the core java course in 5 parts:
Part A:Introduction part B:Overview of java platform part C:Java language fundamentals part D:Object oriented programming Basic terminology part E:Object Oriented programming FeaturesTuesday 30 May 2017
C# Array Class
Properties of Array Class: Let me explain properties of Array Class with the help of below example: using System; public class Test { public static void Main() { int[] arr =new int[4]; for(int i=0;i<4;i++){ arr[i]=i; } for(int i=0;i<4;i++){ Console.Write(arr[i]+" "); } Console.WriteLine(); //properties of array class like mentioned below //length Console.WriteLine(arr.Length); //LongLength Console.WriteLine(arr.LongLength); //Rank Console.WriteLine(arr.Rank); //is readonly Console.WriteLine(arr.IsReadOnly); //if fixed size Console.WriteLine(arr.IsFixedSize); } } Output: 0 1 2 3 4 4 1 False True
Methods of array class Let me explain some of the important methods with the help of below example: using System; public class Test { public static void Main() { int[] arr =new int[4]; for(int i=0;i<4;i++){ arr[i]=i; } for(int i=0;i<4;i++){ Console.Write(arr[i]+" "); } Console.WriteLine(); //methods of array class like mentioned below //Reverse Array.Reverse(arr); for(int i=0;i<4;i++){ Console.Write(arr[i]+" "); } Console.WriteLine(); //Sort Array.Reverse(arr); for(int i=0;i<4;i++){ Console.Write(arr[i]+" "); } Console.WriteLine(); //GetLength() it will take argument as which dimension you want length //means 0: one D // 1 : find the length of 2d columns Console.WriteLine(arr.GetLength(0)); //GetType() Console.WriteLine(arr.GetType()); } } Output: 0 1 2 3 3 2 1 0 0 1 2 3 4 System.Int32[] I hope now you have idea about array in C#. If still you have any doubt please comment below . I will try to explain with the help of example . Thanks
Array Tutorials In C#
Q) What is an array? Ans) An array is collection of elements of same type. Array consists of Contiguous memory location. Example: Let say you have an array “int number[]” of size n; Then array index will be from 0 to n-1. Number[0] to Number[n-1]
Q) How to declare an array? Ans) In C# we declare array as follows: Datatype[] name_of_array; : Correct syntax Datatype name_of_array[] : Incorrect Syntax Example : Code 1: using System; public class Test { public static void Main() { int arr[]; } } Output: prog.cs(7,12): error CS1525: Unexpected symbol `[', expecting `,', `;', or `=' Compilation failed: 1 error(s), 0 warnings Code 2: using System; public class Test { public static void Main() { int[] arr; } } OutPut: Compiled successfully.
Q) Initialize the array? Ans): Let me write one line : Int[] arr; What this line represent? Ans: It will declare an array but it will not initialize the array into memory. Int[] arr = new int[10]; This line will initialize array using new keyword. Because array is reference type so new keyword is used to create instance of array.
Q) How to assign values to array? Ans:) there are many ways to assign values of an array Method 1: Int[] number={1,2,3,4,5,6,67}; Method 2: Int[] number = new int[] {1,2,3,4,5,6}; Method 3: Int[] number = new int[5] {1,2,3,4,5}; Method 4: Int[] number= new int[2]; number[0]=1; number[1]=2; Let me explain all these methods with the help of one example: using System; public class Test { public static void Main() { //int[] arr = new int[3]; int[] arr= new int[3]; arr[0]=1; arr[1]=2; arr[2]=3; for(int i=0;i<3;i++){ Console.Write(arr[i]+" "); } Console.WriteLine(); //int[] arr={4,5,6}; int[] arr_one={4,5,6}; for(int i=0;i<3;i++){ Console.Write(arr_one[i]+" "); } Console.WriteLine(); //int[] arr=new int[]{3,5,7}; int[] arr_two=new int[]{3,5,7}; for(int i=0;i<3;i++){ Console.Write(arr_two[i]+" "); } Console.WriteLine(); //int[] arr=new int[]{3,5,7}; int[] arr_three=new int[3]{3,5,8}; for(int i=0;i<3;i++){ Console.Write(arr_three[i]+" "); } Console.WriteLine(); } } Output: 1 2 3 4 5 6 3 5 7 3 5 8
C# array Types: 1. Multidimensional array 2. Jagged Arrays MultiDimensional Array : array in which we have a matrix of size m*n, where m is the number of rows and n is the number of columns. Let me explain with the help of below code: using System; public class Test { public static void Main() { int[,] arr =new int[3,4]; for(int i=0;i<3;i++){ for(int j=0;j<4;j++){ arr[i,j]=i+j; } } for(int i=0;i<3;i++){ for(int j=0;j<4;j++){ Console.Write(arr[i,j]+" "); } Console.WriteLine(); } } } Output: 0 1 2 3 1 2 3 4 2 3 4 5 Explanation: 1) Line : int[,] arr =new int[3,4]; It will declare and initialize the array in memory. It means that we declare an two array having 3 number of rows and 4 number of columns. Let me explain with the help of one more code: using System; public class Test { public static void Main() { int[,] arr =new int[3,4]{{1,2,3,4},{5,6,7,8},{9,10,11,12}}; for(int i=0;i<3;i++){ for(int j=0;j<4;j++){ Console.Write(arr[i,j]+" "); } Console.WriteLine(); } } } Output: 1 2 3 4 5 6 7 8 9 10 11 12 Explanation: In this example we are initializing the 2D array of size 3*4
Jagged Arrays Array in which the number of columns in each row may vary. Let me explain with the help of below code: using System; public class Test { public static void Main() { int[][] arr =new int[3][]; for(int i=0;i<3;i++){ arr[i]= new int[4]; } for(int i=0;i<3;i++){ for(int j=0;j<4;j++){ arr[i][j]=i+j; } } for(int i=0;i<3;i++){ for(int j=0;j<4;j++){ Console.Write(arr[i][j]+" "); } Console.WriteLine(); } } } Output: 0 1 2 3 1 2 3 4 2 3 4 5 Explanation: 1) In the above example we can change the number of column based on our requirement. 2) But here we are taking number of column as 4 for all three rows. Let me explain one more code which has different columns based on rows. ode: using System; public class Test { public static void Main() { int[][] arr =new int[3][]; for(int i=0;i<3;i++){ arr[i]= new int[i+1]; } for(int i=0;i<3;i++){ for(int j=0;j<=i;j++){ arr[i][j]=i+j; } } for(int i=0;i<3;i++){ for(int j=0;j<=i;j++){ Console.Write(arr[i][j]+" "); } Console.WriteLine(); } } } Output: 0 1 2 2 3 4 Explanation: So here we can see that every row has different number of columns. If still you have any doubt please comment below.
Monday 29 May 2017
How To Create First Xamarin.Android Project
How to create a project in Xamarin.Android:
New Project->Android->Blank App->name:of App -> OK
How to Create a Android Emulator(Virutal Device)
Goto Tools ->Android-> Android Emulator Manager-> Create-> Example : Let me create One device as follows details ->Avd Name : devAvd -> Devide : Nexus S ->Target : Android 4.4.2 –API Level 19 -> CPU/ABI : ARM (armeabi-v7a) ->Skin : No skin ->front Camera : None -> Back Camera : None ->Memory Options : RAM : 1024 ->Internal Storage : 500 Click OK
Then you can simply Deploy your project using Emulator.
Complete Installation of Xamarin.Android with Virtual Studio 2015
You have to install all the below step by step: 1. Xamarin.Android : 2. Android SDK 3. Android NDK(x64) 4. GTK# 5. Xamarin Studio 6. Xamarin for Visual Studio
Please Find the download Link:
How to install Xamarin with VS 2015
While Installation Choose options: as clicked in the below two pictures
1.Select C#/.Net(Xamarin) under cross platform mobile app development 2. Select Android SDK(3rd party), Adroid SDK Setup(Api Level 19 and 21) , Java SE development kit
Thursday 25 May 2017
Exception Handling in C#
Exception Handling in C# Q 1) you all have doubt that what is exception and what is exception handling? Before explaining that please have a look at below code: Code1: using System; namespace main_class{ class MainClass{ static void Main(string[] args){ Console.WriteLine("hi this is main function start"); int a=10; int b=0; int div_result; div_result=a/b; Console.WriteLine("{0}",div_result); Console.WriteLine("hi this is main function end"); } } } Output of code is: hi this is main function start Unhandled Exception: System.DivideByZeroException: Division by zero at main_class.MainClass.Main (System.String[] args) [0x00000] in <filename unknown>:0 [ERROR] FATAL UNHANDLED EXCEPTION: System.DivideByZeroException: Division by zero at main_class.MainClass.Main (System.String[] args) [0x00000] in <filename unknown>:0 Explanation: .You are trying to divide number a by 0, which leads to infinity result. .Which is undefined number. .So program execution will stop at that point only. .Means it will not execute further instructions of the program. .That’s why we are not getting the output of the last two console statements. Code 2: using System; namespace main_class{ class First{ public void fun(){ Console.WriteLine("hi this is the fun() method of First class"); } } class MainClass{ static void Main(string[] args){ Console.WriteLine("hi this is main function start"); First obj = new First(); obj=null; obj.fun(); Console.WriteLine("hi this is main function end"); } } } Output of above code is: hi this is main function start Unhandled Exception: System.NullReferenceException: Object reference not set to an instance of an object at main_class.MainClass.Main (System.String[] args) [0x00000] in <filename unknown>:0 [ERROR] FATAL UNHANDLED EXCEPTION: System.NullReferenceException: Object reference not set to an instance of an object at main_class.MainClass.Main (System.String[] args) [0x00000] in <filename unknown>:0 Explanation: .In this code you make object of class First as “null” .Then you are calling fun() method using that object, which is null .so here that object reference is not set to instance of an object .So we will get “NullReferenceException” .Once we will get line obj.fun() our program will stop there only, it will not execute further instructions. Conclusion of above two codes: So both the errors are coming at runtime(during the execution of program) so these are known as exceptions. And Techniques used to handle such kind of exceptions are known as “Exception Handling”. Q 2) What is Exception? Ans ) Let say your program is running and then suddenly you get an error, so this error is known as Exception. In Terms of Definition : “Problem arises during execution of a program is known as Exception.” Q 3) What is Exception Handling? Ans) Process of handling exception is known as exception handling. Q 4) How to use exception handling? Ans: C# exception handling is build upon four keywords: try, catch, finally, throw Let me explain everything with the help of code, I think it will be easy for all of you to understand: Code: using System; namespace main_class{ class MainClass{ static void Main(string[] args){ Console.WriteLine("hi this is main function start"); int a=10; int b=0; try{ int div_result; div_result=a/b; Console.WriteLine("{0}",div_result); } catch(DivideByZeroException e){ Console.WriteLine("you are dividing by zero please re enter the number b"); } catch(NullReferenceException e){ Console.WriteLine("ypu are using a null reference object"); } finally{ Console.WriteLine("It will always execute whether exception will occur or not"); } Console.WriteLine("hi this is main function end"); } } } Output: hi this is main function start you are dividing by zero please re enter the number b hi this is main function end Explanation: 1. Try block: it includes the portion of code where there is a possibility of getting exception. 2. Catch block: . We can write multiple catch block after try . out of those catch block based on the type of exception it will select which catch will handle exception. 3. After executing catch block it will start executing further instruction means our program execution will not stop. 4. finally{} block will always execute whether exception will generate or not. Throw Block Code: using System; namespace main_class{ class MainClass{ static void Main(string[] args){ Console.WriteLine("hi this is main function start"); int a=10; int b=0; try{ int div_result; if(b==0){ throw (new DivideByZeroException("you are dividing by zero please re enter the number b")); } //div_result=a/b; //Console.WriteLine("{0}",div_result); } catch(DivideByZeroException e){ //Console.WriteLine("you are dividing by zero please re enter the number b"); } catch(NullReferenceException e){ Console.WriteLine("ypu are using a null reference object"); } finally{ Console.WriteLine("It will always execute whether exception will occur or not"); } Console.WriteLine("hi this is main function end"); } } } Output: hi this is main function start It will always execute whether exception will occur or not hi this is main function end Explanation: . Throw keyword is used to throw some exception whenever we want or whenever we have the possibility of getting exception, so there we can throw exception. .You can refer to above code for more clarification. Some Important Concept regarding Exception Handling Note1: We can write our own exception class but we have to inherit that class From “Exception” class. And 1 argument constructor is mandatory to write in that class. Please check the below code for more clarification: Code: using System; namespace main_class{ class DivideZeroException: Exception{ public DivideZeroException(string message):base(message){ } } class MainClass{ static void Main(string[] args){ Console.WriteLine("hi this is main function start"); int a=10; int b=0; try{ int div_result; if(b==0){ throw (new DivideZeroException("you are dividing by zero please re enter the number b")); } //div_result=a/b; //Console.WriteLine("{0}",div_result); } catch(DivideZeroException e){ Console.WriteLine("you are dividing by zero please re enter the number b"); } catch(NullReferenceException e){ Console.WriteLine("ypu are using a null reference object"); } finally{ Console.WriteLine("It will always execute whether exception will occur or not"); } Console.WriteLine("hi this is main function end"); } } } Output: hi this is main function start you are dividing by zero please re enter the number b It will always execute whether exception will occur or not hi this is main function end
Subscribe to:
Posts (Atom)
-
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 ...
-
HII guys this is totally geometry based problem there is nothing to code just use formula LOGIC::how to find centroid of a polygon u c...